Overview
Expenses are divided into two parts:
- „expenseCategory“ – Provides the necessary framework conditions, such as currency. It is used to distinguish between expense types.
- „expense“ – The expense element containing all relevant data.
If you want to create a new expense element, you must provide the ID of the expense category. Otherwise, the API will return an error. You can manage this in your system under „Receipt types“.
ExpenseCategory
/api/expensecategory
This endpoint only supports GET requests and is for informational purposes only. It can be used to search for expense category with the type you looking for.
Field | Datatype | Description |
---|---|---|
name | string | name of the category |
internalName | string | internal name of the category |
description | string | Description |
active | boolean | is it usable |
type | enum | expense type |
currency | number | Currency-ID |
defaultPaymentMethod | enum | Default payment method |
defaultVehicle | enum | Default vehicle method |
/api/expensecategory/id
{
"_id": 11582821,
"internalName": "Fahrtkosten",
"type": {
"caption": "Mileage expense",
"href": "http://localhost:8083/api/enum/expensetype/POSITIONTYPE_TRAVELEXPENSE",
"value": "POSITIONTYPE_TRAVELEXPENSE",
"optionsUrl": "http://localhost:8083/api/enum/expensetype"
},
"active": true,
"name": "Fahrtkosten",
"description": null,
"currency": {
"caption": "EUR",
"title": "currency",
"href": "http://localhost:8083/api/currency/8627968",
"value": 8627968,
"idKey": "105"
},
"defaultPaymentMethod": {
"href": null,
"value": null,
"optionsUrl": "http://localhost:8083/api/enum/paymentmethod"
},
"defaultVehicle": {
"caption": "Private car",
"href": "http://localhost:8083/api/enum/vehicle/PRIVATE",
"value": "PRIVATE",
"optionsUrl": "http://localhost:8083/api/enum/vehicle"
}
}
You can also retrieve the ID via your system. To do this, edit an expense category and enable debug mode. Press CTRL + # or CTRL + ^ on your keyboard. Then, copy the ID value.
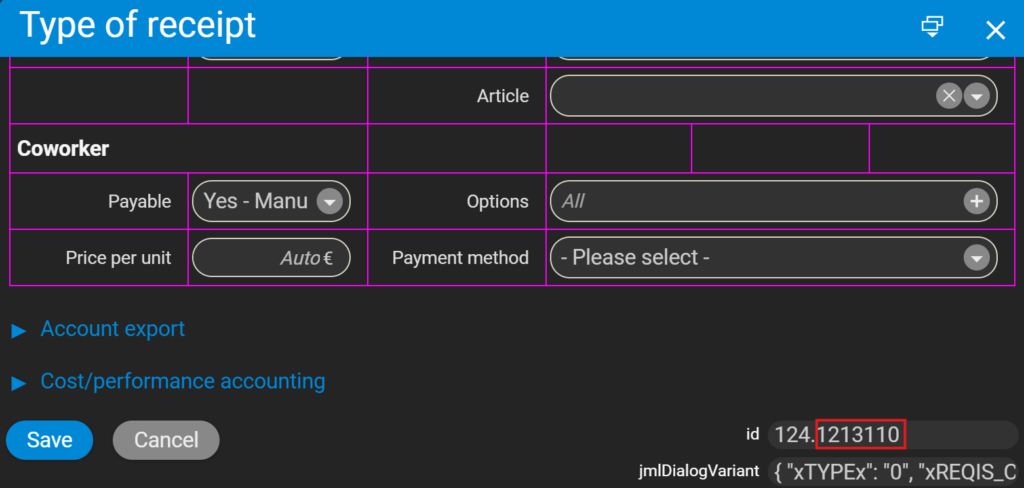
Create and obtain expenses
/api/expense
Through this endpoint, the following entities can be created:
Receipt, Cost invoice, Mileage expense, Material, Provision, Meal expense, Overtime payment and Supplement.
Fields marked with * are mandatory and required for element creation.
Amounts that are not marked as mandatory fields are calculated by the system. You can overwrite them, but there is a risk that they will be overwritten again when manual editing an entry in the system.
For the billable options (externalBillable, projectBillable, and coworkerBillable), the value of the expense category is used as a fallback. The automation adds the amount – analogous to the system – whenever ‚Billable‘ is not ‚NO‘. If you want different values, you must specify them manually.
Here you can find an overview of all API fields:
Field | Datatype | Description |
---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used |
number | string | Number of the voucher (Generated automatically) |
voucherNumber | string | Number of the original voucher. |
description | string | Description |
type | enum | Expense type (travel expense, provision etc.). Determined by the cost category. |
expenseCategory | number | ID of the expense category |
coworker | number | Worker-ID |
project | number | Project-ID |
customer | number | Customer-ID |
financeRecordID | number | Invoice-ID |
quantity | number | Amount (For calculations with base price) |
voucherCurrencyFactor | number | Currency factor of the voucher (Generated automatically) |
voucherVat | number | Vat of the voucher |
voucherCurrency | number | Currency-ID |
voucherNetto | number | Net (Voucher currency) |
voucherGross | number | Gross (Voucher currency) |
voucherPrivateShare | number | Worker private share. Will be deducted from the reimbursement amount (Voucher currency). |
relevantNetto | number | Net amount (Mandant Currency) |
externalBillable | enum | Billable of Organization |
externalNetto | number | Billable amount for organization (Mandant Currency) |
projectBillable | enum | Billable of project |
projectNetto | number | Billable amount for project (Mandant currency) |
coworkerBillable | enum | Billable of worker |
coworkerNetto | number | Billable amount for worker (Mandant currency) |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) |
startLocation | string | start location |
finishLocation | string | finish location |
distance | number | distance in kilometres |
overtime | number | Overtime in minutes |
vehicle | enum | Vehicle type (private / company) |
paymentMethod | enum | Payment method (private / company) |
containsBreakfast | boolean | Contains breakfast |
containsLunch | boolean | Contains lunch |
containsDinner | boolean | Contains dinner |
travelExpense | number | Travel-ID |
time | enum | Time for meal expense |
isTaxMandatory | boolean | Mandatory taxation |
costcenterOverride | number | Cost center ID |
costunitOverride | number | Cost unit ID |
costtypeOverride | number | Cost type ID |
costunitAuto | number | Cost unit ID (From expense category) |
costtypeAuto | number | Cost type ID (From expense category) |
costcenterAuto | number | Cost center ID (From expense category) |
Provision
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
financeRecordID | number | Invoice-ID | |
voucherCurrency | number | Currency-ID | |
* | voucherNetto | number | Net (Voucher currency) |
relevantNetto | number | Net amount (Mandant Currency) | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-01",
"description": "Provision",
"expenseCategory": 176370223,
"coworker": 54967,
"financeRecordID": 1926724,
"voucherCurrency": 8627969,
"voucherNetto": 100,
"coworkerBillable": "YES_MANUAL",
}
Overtime
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
coworkerBillable | enum | Billable of worker | |
* | coworkerNetto | number | Billable amount for worker (Mandant currency) |
* | overtime | number | Overtime in minutes |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
At least one value must be provided for either „coworkerNetto“ or „overtime“.
{
"date": "2025-03-07",
"description": "overtime",
"expenseCategory": 174025224,
"coworker": 54967,
"coworkerBillable": "YES_MANUAL",
"overtime": 60
}
Mileage expense
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
voucherNumber | string | Number of the original voucher. | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
voucherNetto | number | Net (Voucher currency) | |
relevantNetto | number | Net amount (Mandant Currency) | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) | |
startLocation | string | start location | |
finishLocation | string | finish location | |
* | distance | number | distance in kilometres |
* | vehicle | enum | Vehicle type (private / company) |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-03",
"description": "travel",
"expenseCategory": 11582821,
"coworker": 54967,
"distance": 100,
"vehicle": "PRIVATE"
}
Material expense
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
voucherNumber | string | Number of the original voucher. | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
* | quantity | number | Amount (For calculations with base price) |
relevantNetto | number | Net amount (Mandant Currency) | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) | |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-07",
"expenseCategory": 15478132,
"coworker": 54967,
"quantity": 100,
"coworkerBillable": "YES_MANUAL",
}
Expense voucher
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
voucherNumber | string | Number of the original voucher. | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
* | quantity | number | Amount (For calculations with base price) |
voucherCurrency | number | Currency-ID | |
* | voucherNetto | number | Net (Voucher currency) |
* | voucherGross | number | Gross (Voucher currency) |
voucherPrivateShare | number | Worker private share. Will be deducted from the reimbursement amount (Voucher currency). | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) | |
* | paymentMethod | enum | Payment method (private / company) |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
If both „voucherNetto“ and „voucherGross“ are null, the base price from the category will be used. If no base price is defined, an error will be returned. If only „voucherGross“ is provided, „voucherNetto“ will be calculated.
{
"date": "2025-03-07",
"expenseCategory": 12199867,
"coworker": 54967,
"quantity": 2,
"voucherCurrency": 8627968,
"voucherNetto": 200,
"voucherPrivateShare": 10,
"paymentMethod": "PRIVATE"
}
Meal expense
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
relevantNetto | number | Net amount (Mandant Currency) | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) | |
* | containsBreakfast | boolean | Contains breakfast |
* | containsLunch | boolean | Contains lunch |
* | containsDinner | boolean | Contains dinner |
travelExpense | number | Travel-ID | |
* | time | enum | Time for meal expense |
isTaxMandatory | boolean | Mandatory taxation | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-10",
"expenseCategory": 69707469,
"coworker": 54967,
"containsBreakfast": true,
"containsLunch": true,
"time": "RATE_24H",
"containsDinner": false,
"taxMandatory": false,
}
Supplement
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
* | voucherNetto | number | Net (Voucher currency) |
relevantNetto | number | Net amount (Mandant Currency) | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-14",
"expenseCategory": 185792490,
"coworker": 54967,
"voucherNetto": 100,
"coworkerBillable": "YES_MANUAL"
}
Expense invoice
Field | Datatype | Description | |
---|---|---|---|
date | string | Date of the voucher in ISO format (YYYY-MM-DD). As default the current day is used | |
number | string | Number of the voucher (Generated automatically) | |
description | string | Description | |
* | expenseCategory | number | ID of the expense category |
* | coworker | number | Worker-ID |
project | number | Project-ID | |
customer | number | Customer-ID | |
* | financeRecordID | number | Invoice-ID |
voucherPrivateShare | number | Worker private share. Will be deducted from the reimbursement amount (Voucher currency). | |
externalBillable | enum | Billable of Organization | |
externalNetto | number | Billable amount for organization (Mandant Currency) | |
projectBillable | enum | Billable of project | |
projectNetto | number | Billable amount for project (Mandant currency) | |
coworkerBillable | enum | Billable of worker | |
coworkerNetto | number | Billable amount for worker (Mandant currency) | |
coworkerNettoTaxfree | number | tax free billable amount for worker (Mandant currency) | |
* | paymentMethod | enum | Payment method (private / company) |
travelExpense | number | Travel-ID | |
costcenterOverride | number | Cost center ID | |
costunitOverride | number | Cost unit ID | |
costtypeOverride | number | Cost type ID |
{
"date": "2025-03-13",
"expenseCategory": 185767407,
"coworker": 54967,
"financeRecordID": 185797405,
"paymentMethod": "PRIVATE",
}